Let's figure out how to read port input data on an AVR processor using C.
Connect PortB To Switches
Remember how we connected PORTA to the LEDS? Now we are going to connect PORTB to the SWITCHES. Just be sure that you connect pin 1 of PORTB also known as PB0 toSW0 by orienting the cable so that the red stripe is on the correct side on both headers.
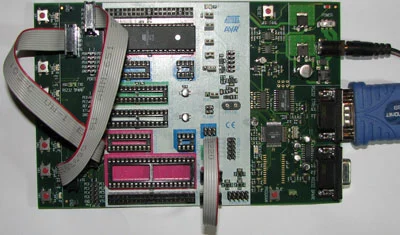
AVR Port Input
Before you can read input from a port on an AVR microcontroller you need to configure the pins that you are going to read to be input pins.
In our AVR Port Output Using C guide we talked about the Data Direction Registers. Review that guide if you are not familiar with the concept.
You should also write 0's out to PORTB to ensure that it is in high impedance mode. If PORTB has 1's in it, then the AVR will source current if pulled externally low. Let's set ourPORTB up as an input port.
// setup PORTB data direction as an input
DDRB = 0;
// make sure it is high impedance and will not source
PORTB = 0;
Map Switches to LED's
For a really simple test application, let's map the PORTB switch inputs to the PORTA lights. This way, any time you press a button on the STK500, the corresponding LED will turn on. Remember that the LEDs on the STK500 are wired in reverse logic.
So are the switches. This means that an open switch is feeding a 1 in to the corresponding pin, which will send a 1 out to the corresponding LED and that LED will be off.
When you press a switch a 0 will be read in, which is sent out PORTA and the LED will light up. To read the input from a pin, you read the PINx value. In this case, we are going to read PINB and map it to PORTA, like this.
PORTA = PINB;
Do It In The Loop
If you just put the above instruction in your program outside of a loop, then it would only happen once, and your PORTA lights will reflect the state of your PINB inputs at the time you turn on the STK500.
To make the LEDs mimic the switches for ever, put the above line inside your main loop. Below is the complete code to map PINB switches to PORTA LEDs.
// ********************************************************************************
// Includes
// ********************************************************************************
#include <avr/io.h>
#include <stdbool.h>
// ********************************************************************************
// Main
// ********************************************************************************
int main(void)
{
ÃÂ ÃÂ ÃÂ ÃÂ // configure PORTA as output
ÃÂ ÃÂ ÃÂ ÃÂ DDRA = 0xFF;
ÃÂ ÃÂ ÃÂ ÃÂ // configure PORTB as input
ÃÂ ÃÂ ÃÂ ÃÂ DDRB = 0;
ÃÂ ÃÂ ÃÂ ÃÂ // make sure PORTB is high impedance and will not source
ÃÂ ÃÂ ÃÂ ÃÂ PORTB = 0;
ÃÂ ÃÂ ÃÂ ÃÂ // main loop
ÃÂ ÃÂ ÃÂ ÃÂ while (true)
{
ÃÂ ÃÂ ÃÂ ÃÂ ÃÂ ÃÂ ÃÂ ÃÂ // map PINB switches to PORTA
ÃÂ ÃÂ ÃÂ ÃÂ ÃÂ ÃÂ ÃÂ ÃÂ PORTA = PINB;
}
}
You can download the complete source code here.
Inverting the Values
If you want to invert the lights so that they are all on, and only turn off when you press a switch, simply replace PORTA = PINB; with this:
PORTA = ~PINB;
The tilde ~ will invert the inputs from PINB before mapping them out to PORTA.
Making Decisions
Now that we can read the input from an AVR pin, we can start doing some AVR Port Input Decision Making. Or head back to our index of AVR Guides here.