Sometimes you need to swap one color in an image with another color.
How to Swap a Color in an Image with C#
In C# we do this by first opening the image up from file and loading it into a Bitmap object. Once we have it in a Bitmap we can iterate over the x and y values looking at 1 pixel at a time. Each time we look at a pixel we can decide if we want to swap it with a new color based on it's current color. After that we can save it to a new file, display it on screen, or both. The color below does the following:
- Open an image file into a Bitmap object
- Iterate over the pixels replacing all red pixels with yellow
- Display the modified image on a PictureBox on a form
- Save the modified image as a lossless PNG
Example Code in C#
Here's the code.
// swap one color with another
private static void SwapColor(Bitmap bmp, Color oldColor, Color newColor)
{
var lockedBitmap = new LockBitmap(bmp);
lockedBitmap.LockBits();
for (int y = 0; y < lockedBitmap.Height; y++)`
{
for (int x = 0; x < lockedBitmap.Width; x++)
{
if (lockedBitmap.GetPixel(x, y) == oldColor)
{
lockedBitmap.SetPixel(x, y, newColor);
}
}
}
lockedBitmap.UnlockBits();
}
Swap Color Results
Here's the input and output images so that you can see exactly what happened. `
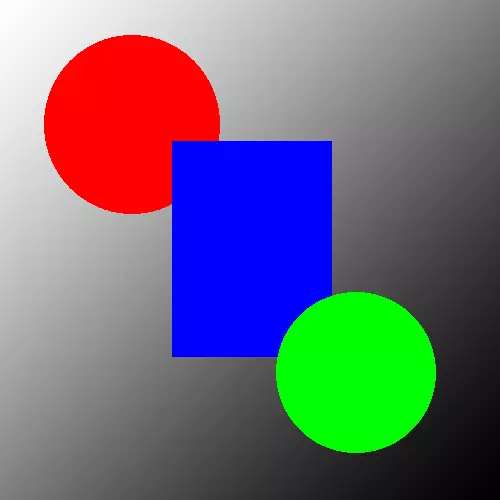
After running the above code on this image, here's the output image that has red swapped yellow.
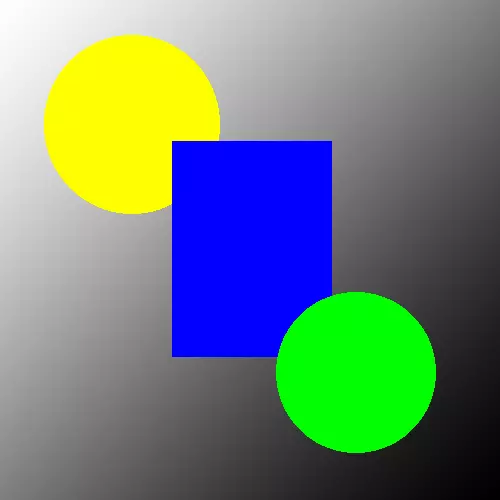
Important Notes about LockBitmap
For the most part this is pretty simple code. The real "meat-and-potatoes" part is the function SwapColor. The first thing it does is lock the Bitmap object. In order to do this you need the LockBitmap class, which I explain on the How to Load an Image guide. If you need the LockBitmap class you can find it there.
Image Transperancy
After locking the Bitmap all we have to do is iterate over the width and height checking each pixel for a color match. Notice that the Color object includes an alpha, or transparency, channel. If you're not sure what that means you might want to read our article about C# Color Objects.
C# Reference Types
You may have noticed that the main function SwapColor does not return a result. Instead, it operates on the Bitmap object that was passed in. The Bitmap object is called a Reference Type in C#. This means that when you pass it to a function you are passing a reference to it as opposed to a copy. This makes it so that you do not have to create a second copy in RAM to modify and hand back to the calling function.
C# Image Processing Guides
If you like this guide then you might want to read some of our other C# Image Processing Guides.