If you need to change the size of an Image without changing the ratio of X to Y then this function should do what you want.
Function to Scale a Bitmap in C#
The input parameters maxWidth and maxHeight define the largest values that you want to get back out of the image after it has been scaled.
Here's the code:
static Bitmap ScaleImage(Bitmap bmp, int maxWidth, int maxHeight)
{
var ratioX = (double)maxWidth / bmp.Width;
var ratioY = (double)maxHeight / bmp.Height;
var ratio = Math.Min(ratioX, ratioY);
var newWidth = (int)(bmp.Width * ratio);
var newHeight = (int)(bmp.Height * ratio);
var newImage = new Bitmap(newWidth, newHeight);
using(var graphics = Graphics.FromImage(newImage))
graphics.DrawImage(bmp, 0, 0, newWidth, newHeight);
return newImage;
}
Results of Scaling an Image in C#
Here is a test image that we loaded into the scale function.
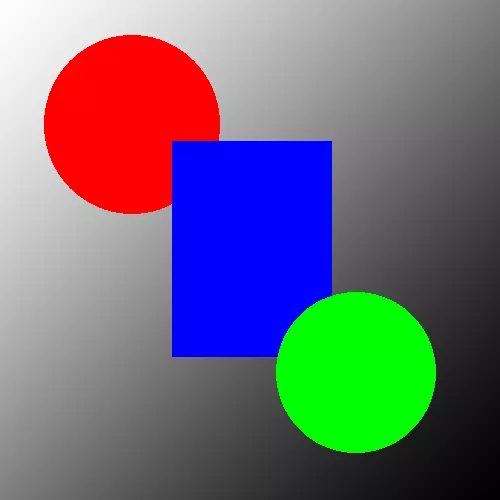
And here it is after calling for a maxWidth of 5000 and a maxHeight of 200.
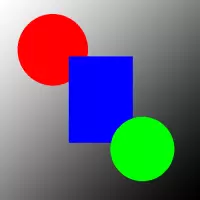
You can see that the maxHeight of 200 was obeyed and the image was scaled while maintaining a proper aspect ratio.
Other Image Processing Guides
You may want to check out some of our other Programming Guides in C#.